Dictionary instead of switch or if statements
Conditions are a basic concept of programming but best practices suggest to avoid excessive usage of if statements. They say that if conditions make more complex and could buggy our applications because too many if-else can cause large numbers of condition branches. We cannot fully remove if statements, but we can reduce their usage.
Do you need to map datas from A to B without more logic? Is it a simple key/value mapping? Set up a dictionary data structure instead of switch or if-else ladder.
Suppose we need to manage the enum of Pokemon types and map for some reason each type to a base hit point value. Let’s write using switch, if-else and dictionary structure, with some semplifications:
If statement
public int GetHitPoint(PokemonType value)
{
var point = 0;
if(value == PokemonType.NORMAL)
{
point = 1;
}
else if(value == PokemonType.GRASS)
{
point = 2;
}
else if(value == PokemonType.ELECTRIC)
{
point = 3;
}
else if(value == PokemonType.WATER)
{
point = 4;
}
else if(value == PokemonType.FIRE)
{
point = 5;
}
else if(value == PokemonType.BUG)
{
point = 6;
}
[...]
return point;
}
Switch statement
public int GetHitPoint(PokemonType value)
{
var point = 0;
switch(value)
{
case PokemonType.NORMAL:
point = 1;
break;
case PokemonType.GRASS:
point = 2;
break;
case PokemonType.ELECTRIC:
point = 3;
break;
case PokemonType.WATER:
point = 4;
break;
case PokemonType.FIRE:
point = 5;
break;
case PokemonType.BUG:
point = 6;
break;
[...]
}
return point;
}
Dictionary structure
public int GetHitPoint(PokemonType value)
{
var basePointMapping = new Dictionary<PokemonType, int>
{
{PokemonType.NORMAL, 1},
{PokemonType.GRASS, 2},
{PokemonType.ELECTRIC, 3},
{PokemonType.WATER, 4},
{PokemonType.FIRE, 5},
{PokemonType.BUG, 6}
[...]
};
return basePointMapping[value];
}
Conclusion
As you can see, without any kind of explanation, in term of readability and compactness, dictionaries are the first choice, I wrote less lines, code is clean, easy to refactor. Could be the data structure to consider in these type of simple mappings, remember the KISS principle, you need to avoid a large number of cases in switch/if statements because they are less maintainable.
[EDIT] I have created a repository on this topic, Github repo: https://github.com/apulito/dictionary-instead-statements-v1
Hope this gives some inspiration!
Bye, Alberto
Related Posts
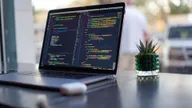
Using Value Tuple
All of us write methods that return one value, string method return a string, int method return an int, but what do you do when you need to return more than one value?
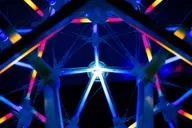
Dictionary instead of switch or if statements v2
In the previous article I talked about how, in my opinion, is possible to improve the code quality by storing keys/values in a dictionary instead of using switch or conditional statements.
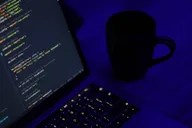
Organize top level solution folders in .NET
There are different ways to create Multi-Project Solution or Single Project solution from scratch, for example using the .NET command-line interface (CLI) or the Visual Studio (VS) GUI